Common Datetime usage with C#
Jan 05 2024 10:21
The DateTime value type represents dates and times with values ranging from 00:00:00 (midnight), January 1, 0001 Anno Domini (Common Era) through 11:59:59 P.M., December 31, 9999 A.D. (C.E.) in the Gregorian calendar.
Time values are measured in 100-nanosecond units called ticks. A particular date is the number of ticks since 12:00 midnight, January 1, 0001 A.D. (C.E.) in the GregorianCalendar calendar. The number excludes ticks that would be added by leap seconds. For example, a ticks value of 31241376000000000L represents the date Friday, January 01, 0100 12:00:00 midnight. A DateTime value is always expressed in the context of an explicit or default calendar.
Initialize a DateTime object
Use constructor
var minValue = new DateTime();
Console.WriteLine(minValue); // 01/01/0001 00:00:00
Console.WriteLine(minValue.Equals(DateTime.MinValue)); // True
// parameters order year, month, day, hour, minute, second
var dateFromNumber = new DateTime(2023, 12, 20, 9, 30, 0);
Console.WriteLine(dateFromNumber); // 20/12/2023 09:30:00
User DateTime class
DateTime date1 = DateTime.Now;
DateTime date2 = DateTime.UtcNow;
DateTime date3 = DateTime.Today;
Console.WriteLine(date1); // 04/01/2024 11:47:28
Console.WriteLine(date2); // 04/01/2024 04:47:28
Console.WriteLine(date3); // 04/01/2024 00:00:00
Parse from string
var dateString = "25/12/2023 18:30:25";
// Parse use default CultureInfo.CurrentCulture
var dateValue = DateTime.Parse(dateString);
// Specific Culture
dateValue = DateTime.Parse(dateString, CultureInfo.CurrentCulture);
// Use format pattern
dateValue = DateTime.ParseExact(dateString, "dd/MM/yyyy HH:mm:ss", CultureInfo.InvariantCulture);
// User convert class
dateValue = Convert.ToDateTime(dateString);
Console.WriteLine(dateValue); // 25/12/2023 18:30:25
Convert class is a utility class, Convert.ToDateTime just call DateTime.Parse() but with a null check and return min value datetime
public static DateTime ToDateTime(string? value)
{
if (value == null)
return new DateTime(0);
return DateTime.Parse(value);
}
Format DateTime object
// Use different Culture to format datetime
Console.WriteLine(dateFromNumber.ToString(CultureInfo.InvariantCulture)); // 12/20/2023 09:30:00
Console.WriteLine(dateFromNumber.ToString(CultureInfo.GetCultureInfo("en-US"))); // 12/20/2023 9:30:00AM
Console.WriteLine(dateFromNumber.ToString(CultureInfo.GetCultureInfo("de-DE"))); // 20.12.2023 09:30:00
// Custom format
Console.WriteLine(dateFromNumber.ToString("dd-MM-yyyy HH:mm:ss")); // 20-12-2023 09:30:00
// C# 6 String interpolation format
Console.WriteLine($"{dateFromNumber:dd-MM-yyyy HH:mm:ss}"); // 20-12-2023 09:30:00
Modify DateTime object
var today = new DateTime(2024, 1, 1, 9, 30, 0);
var yesterday = today.AddDays(-1);
var next30Days = today.AddDays(30);
Console.WriteLine($"{yesterday} - {today} - {next30Days}");
// Result: 31/12/2023 09:30:00 - 01/01/2024 09:30:00 - 31/01/2024 09:30:00
// Another Add function
today.AddSeconds(1);
today.AddMinutes(1);
today.AddHours(1);
today.AddDays(1);
today.AddMonths(1);
today.AddYears(1);
Working with JSON (System.Text.Json)
Serialize
System.Text.Json.JsonSerializer user default ISO-8601 time format, ex: "2022-01-31T13:15:05.2151663-05:00"
var today = new DateTime(2024, 1, 1, 9, 30, 0);
Console.WriteLine(JsonSerializer.Serialize(today)); //"2024-01-01T09:30:00"
To custom the format, we need to register a new JSON converter
class SimpleDateTimeConvert : JsonConverter<DateTime>
{
private readonly string _format;
public SimpleDateTimeConvert(string format)
{
_format = format;
}
public override DateTime Read(ref Utf8JsonReader reader, Type typeToConvert, JsonSerializerOptions options)
{
return DateTime.ParseExact(reader.GetString()!, _format, CultureInfo.InvariantCulture);
}
public override void Write(Utf8JsonWriter writer, DateTime value, JsonSerializerOptions options)
{
writer.WriteStringValue(value.ToString(_format, CultureInfo.InvariantCulture));
}
}
Add the new converter to JsonSerializerOptions and pass these options to Serialize function
var serializerOptions = new JsonSerializerOptions
{
Converters = { new SimpleDateTimeConvert("dd-MM-yyyy HH:mm:ss") }
};
Console.WriteLine(JsonSerializer.Serialize(today, serializerOptions)); //"01-01-2024 09:30:00"
Deserialize
Define simple Order class
class Order
{
public int Id { get; set; }
public DateTime PurchaseDate { get; set; }
}
Deserialize with JsonSerializerOptions
var orderJson =
"""
{
"order" : {
"Id" : 1,
"PurchaseDate" : "01-01-2024 09:30:00"
}
}
""";
var order = JsonSerializer.Deserialize<Order>(orderJson, serializerOptions);
Code example at: Github
Delete comment
Confirm delete comment
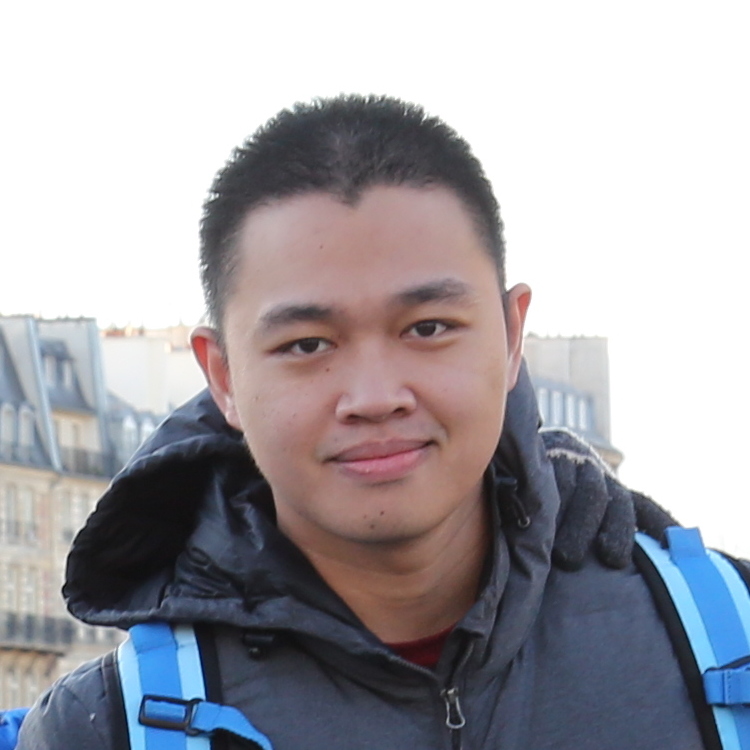
Pham Duc Minh
Da Nang, Vietnam